This is a tip for making sure your devices get turned on regularly (Via BIOS Settings), then you can also turn them off automatically as well (Via Scheduled Task). I have a lot of test devices in my lab, and I like to make sure they turn on at least once a week to check in for inventory and apply updates. I use the built in BIOS “Scheduled Power-On” settings to have it turn on Wednesday mornings each week.
I then have Scheduled tasks that turn the devices in my lab off daily at 6PM. If I want to access them any other day, I either Wake on LAN, or go to my rack and push the power button.
To find the settings on your HP, using HPCMSL, search for “Scheduled Power-On”. By Default, these will have values of “0” and “Disabled”
Get-HPBIOSSettingsList | Select-Object -Property name, path, value | Where-Object {$_.path -match "Scheduled Power-On"}

Once you’ve modified them, they will be set to a specific time, and Enabled for the day.

This computer now shows that the device is scheduled to turn on at 4:30 AM on Wednesday(s)
Using PowerShell, this can be done pretty easily. Here is a breakdown:
Discovery
#Discovery Script:
$Compliance = "Compliant"
$SettingName = 'BIOS Power-On Minute'
$Value = '30'
#-------------------------------------
$BIOSSetting = Get-WmiObject -class "HP_BIOSInteger" -Namespace "root\hp\instrumentedbios" -Filter "Name='$SettingName'"
#Write-Output $BIOSSetting.Value
if ($BIOSSetting.Value -ne $Value){$Compliance = "$($BIOSSetting.Value)"}
$SettingName = 'BIOS Power-On Hour'
$Value = '4'
#-------------------------------------
$BIOSSetting = Get-WmiObject -class "HP_BIOSInteger" -Namespace "root\hp\instrumentedbios" -Filter "Name='$SettingName'"
#Write-Output $BIOSSetting.Value
if ($BIOSSetting.Value -ne $Value){$Compliance = "$($BIOSSetting.Value)"}
$SettingName = 'Wednesday'
$Value = 'Enable'
#-------------------------------------
$BIOSSetting = Get-WmiObject -class "HP_BIOSSetting" -Namespace "root\hp\instrumentedbios" -Filter "Name='$SettingName'"
#Write-Output $BIOSSetting.CurrentValue
if ($BIOSSetting.CurrentValue -ne $Value){$Compliance = "$($BIOSSetting.CurrentValue)"}
$Compliance
This script checks the 3 values I want to set, and if they aren’t what I want, it reports something other than “Compliant”, which is what my CI is looking for.
Default Results for the Script:

After Remediations:

Remediation
#Remediation Script:
$BIOSPW = 'P@ssw0rd'
#Setting 1
$SettingName = 'BIOS Power-On Minute'
$Value = '30'
#-------------------------------------
$BIOS = Get-WmiObject -class "HP_BIOSSettingInterface" -Namespace "root\hp\instrumentedbios"
$BIOSInteger = Get-WmiObject -Class "HP_BIOSInteger" -Namespace "root\hp\instrumentedbios"
$BIOSSetting = Get-WmiObject -class "HP_BIOSSetting" -Namespace "root\hp\instrumentedbios"
If (($BIOSSetting | ?{ $_.Name -eq 'Setup Password' }).IsSet -eq 0)
{
$Result = $BIOS.SetBIOSSetting($SettingName,$Value)
}
elseif (($BIOSSetting | ?{ $_.Name -eq 'Setup Password' }).IsSet -eq 1)
{
$PW = "<utf-16/>$BIOSPW"
$Result = $BIOS.SetBIOSSetting($SettingName,$Value,$PW)
}
#Setting 2
$SettingName = 'BIOS Power-On Hour'
$Value = '4'
#-------------------------------------
$BIOS = Get-WmiObject -class "HP_BIOSSettingInterface" -Namespace "root\hp\instrumentedbios"
$BIOSInteger = Get-WmiObject -Class "HP_BIOSInteger" -Namespace "root\hp\instrumentedbios"
$BIOSSetting = Get-WmiObject -class "HP_BIOSSetting" -Namespace "root\hp\instrumentedbios"
If (($BIOSSetting | ?{ $_.Name -eq 'Setup Password' }).IsSet -eq 0)
{
$Result = $BIOS.SetBIOSSetting($SettingName,$Value)
}
elseif (($BIOSSetting | ?{ $_.Name -eq 'Setup Password' }).IsSet -eq 1)
{
$PW = "<utf-16/>$BIOSPW"
$Result = $BIOS.SetBIOSSetting($SettingName,$Value,$PW)
}
#Setting 3
$SettingName = 'Wednesday'
$Value = 'Enable'
#-------------------------------------
$BIOS = Get-WmiObject -class "HP_BIOSSettingInterface" -Namespace "root\hp\instrumentedbios"
$BIOSInteger = Get-WmiObject -Class "HP_BIOSInteger" -Namespace "root\hp\instrumentedbios"
$BIOSSetting = Get-WmiObject -class "HP_BIOSSetting" -Namespace "root\hp\instrumentedbios"
If (($BIOSSetting | ?{ $_.Name -eq 'Setup Password' }).IsSet -eq 0)
{
$Result = $BIOS.SetBIOSSetting($SettingName,$Value)
}
elseif (($BIOSSetting | ?{ $_.Name -eq 'Setup Password' }).IsSet -eq 1)
{
$PW = "<utf-16/>$BIOSPW"
$Result = $BIOS.SetBIOSSetting($SettingName,$Value,$PW)
}
Exit $Result.Return
This script will leverage WMI to apply the BIOS Settings desired value. Stealing a little code from Mike Terrill, it will check to see if there is a BIOS password, if set, it will use the one you set in the script.
BONUS – Auto Shutdown Scheduled Task via CI
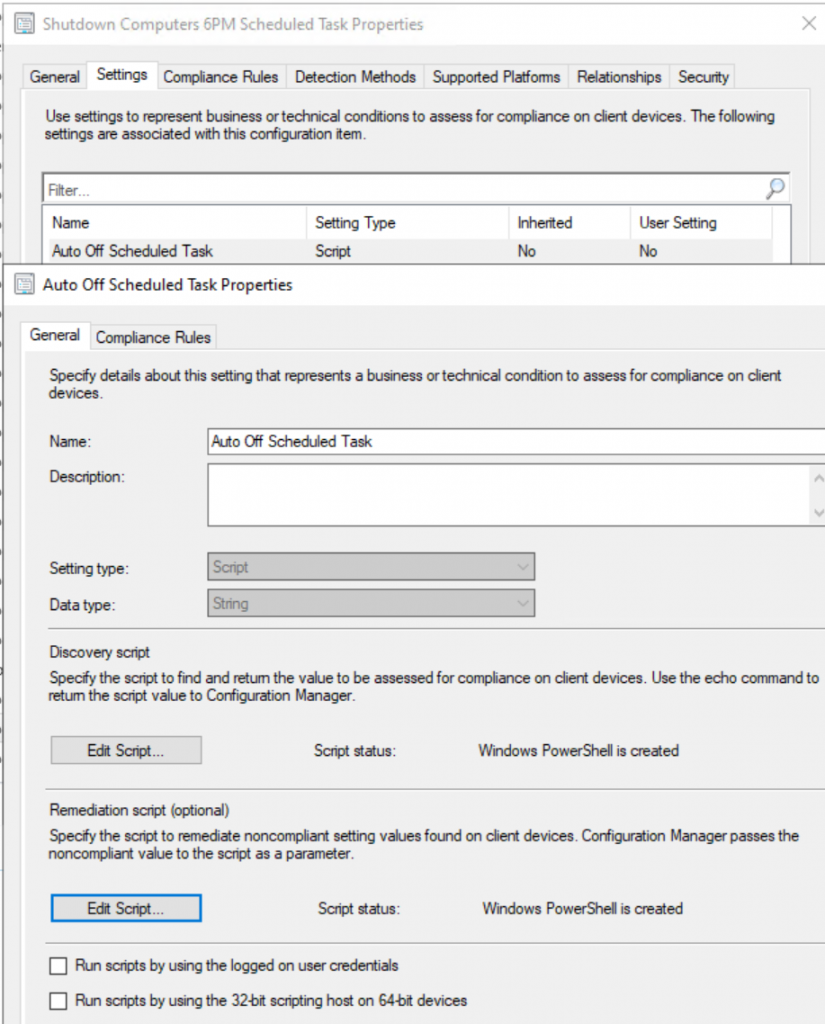
Discovery Script
$GetTask = Get-ScheduledTask -TaskName "Shutdown Computer Daily 6PM" -ErrorAction SilentlyContinue
if (!($GetTask)){
Write-Output "Non-Compliant"
}
else {Write-Output "Compliant"}
Remediation Script
$GetTask = Get-ScheduledTask -TaskName "Shutdown Computer Daily 6PM" -ErrorAction SilentlyContinue
if (!($GetTask)){
$A = New-ScheduledTaskAction -Execute "C:\Windows\System32\WindowsPowerShell\v1.0\powershell.exe" -Argument 'Stop-Computer -Force'
$T = New-ScheduledTaskTrigger -Daily -DaysInterval 1 -At 6PM
$P = New-ScheduledTaskPrincipal "NT Authority\System"
$S = New-ScheduledTaskSettingsSet -AllowStartIfOnBatteries -DontStopIfGoingOnBatteries
$task = New-ScheduledTask -Action $A -Trigger $T -Principal $P -Settings $S
Register-ScheduledTask -TaskName "Shutdown Computer Daily 6PM" -InputObject $Task -Force
}
This is how I keep my lab turning on regularly to get patched, and how I save energy by having them shut off daily.
BONUS 2 – Set BIOS Settings via HP Connect
If you’re using Intune to manage your HP devices, you best be using HP Connect. I’ve been leveraging this to make improve BIOS security (Using HP Sure Admin), easily set BIOS settings, and update BIOS.
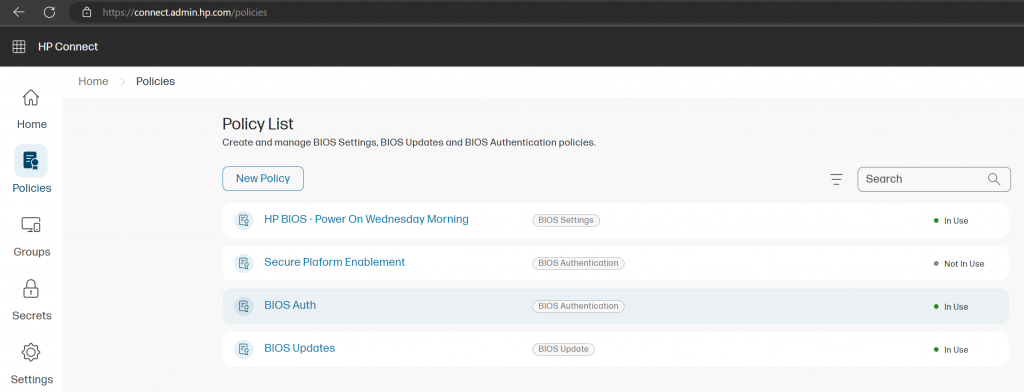
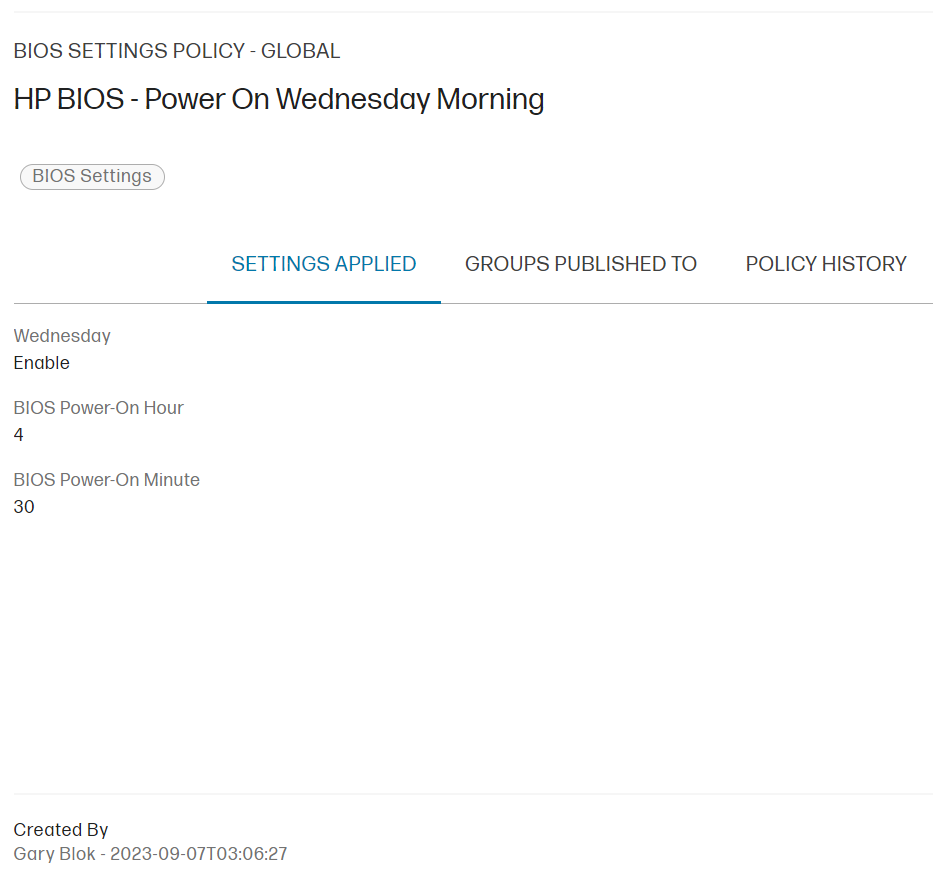
Create the policy and choose the Intune group to deploy to and done. So Simple!